Forms
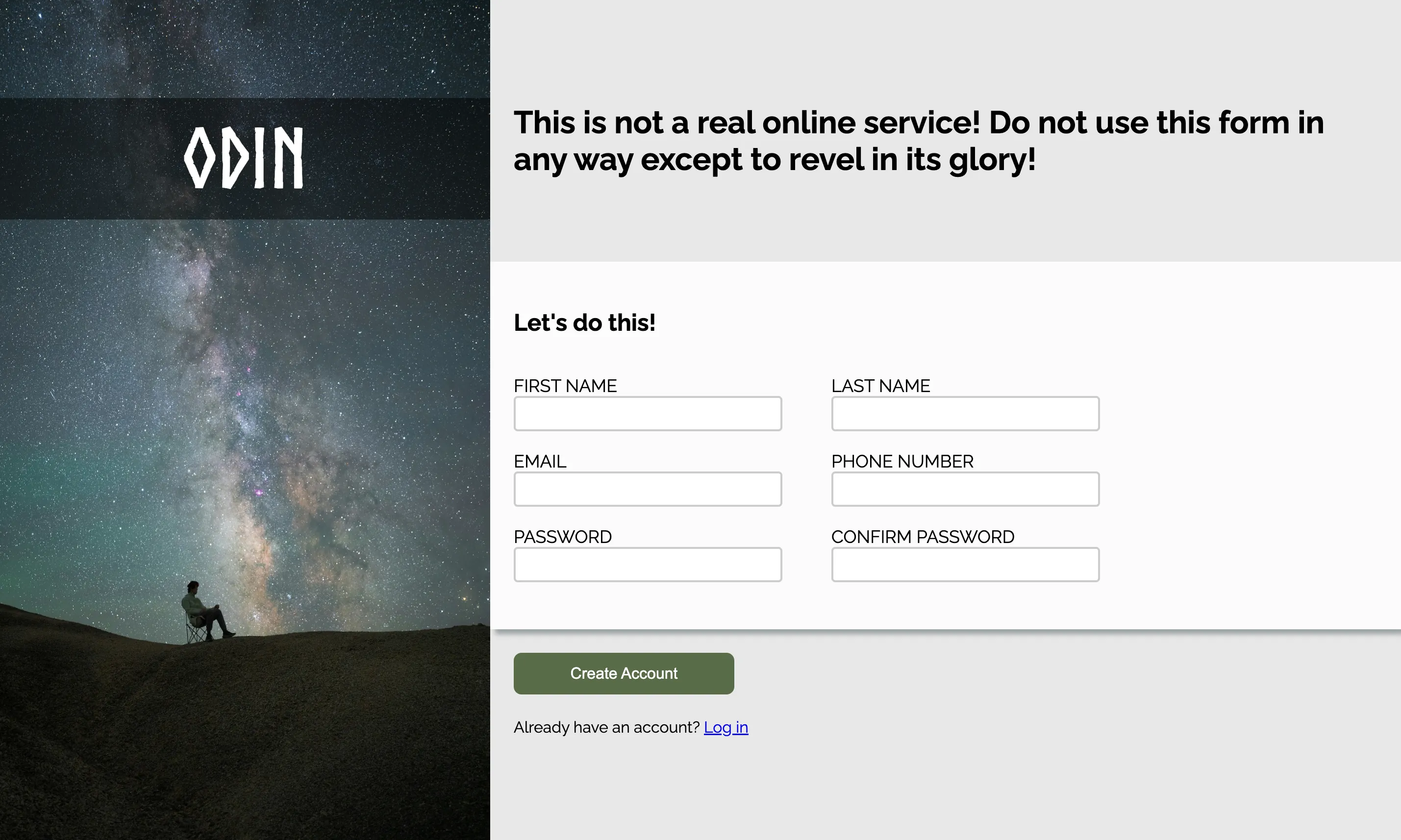
A simple sign-up form template that has form validation.
Overview
This project is a sign-up form that demonstrates various web development concepts using HTML, CSS, and JavaScript. The form includes fields for first name, last name, email, phone number, password, and confirm password. It also includes client-side validation to ensure that the user inputs are correct before submission.
Key Coding Concepts
HTML
- Form Elements: The form is created using the
<form>
element, with various input fields such as<input type="text">
,<input type="email">
,<input type="number">
, and<input type="password">
. - Semantic HTML: The use of semantic elements like
<h2>
,<label>
, and<button>
improves the accessibility and readability of the code.
CSS
- Flexbox: The layout is managed using CSS Flexbox, which allows for flexible and responsive design. Classes like
.sidebar
,.right
,.container
, and.input-group
utilize Flexbox properties. - Custom Fonts: The project imports custom fonts from Google Fonts and uses a local font file (
Norse-Bold.otf
) with the@font-face
rule. - Styling: Various CSS properties are used to style the form, including
background-color
,border
,padding
,margin
, andbox-shadow
.
JavaScript
- Event Listeners: JavaScript is used to add event listeners for form validation. The
blur
event is used for live validation, and thesubmit
event is used for final form validation. - DOM Manipulation: Functions like
showError
andclearError
manipulate the DOM to display or hide error messages and change the border color of input fields. - Validation: The
validateField
function checks for various validation rules, such as required fields, valid email format, minimum password length, and matching passwords.
Client-Side Validation
- Real-Time Feedback: The form provides real-time feedback to the user by validating input fields as they lose focus (on
blur
event). - Error Messages: Custom error messages are displayed below the input fields when validation fails.
- Form Submission: The form prevents submission if any validation errors are present, ensuring that only valid data is submitted.
File Structure
index.html
: The main HTML file containing the structure of the form.styles.css
: The CSS file for styling the form and layout.login.js
: The JavaScript file for handling form validation and user interactions.files/Norse-Bold.otf
: The custom font file used in the project.images/
: A directory containing images used in the project.
How to Run
- Open
index.html
in a web browser. - Fill out the form fields and observe the real-time validation feedback.
- Submit the form to see the final validation check.
Conclusion
This project showcases the integration of HTML, CSS, and JavaScript to create a functional and visually appealing sign-up form with client-side validation.